Streaming payments on Stellar
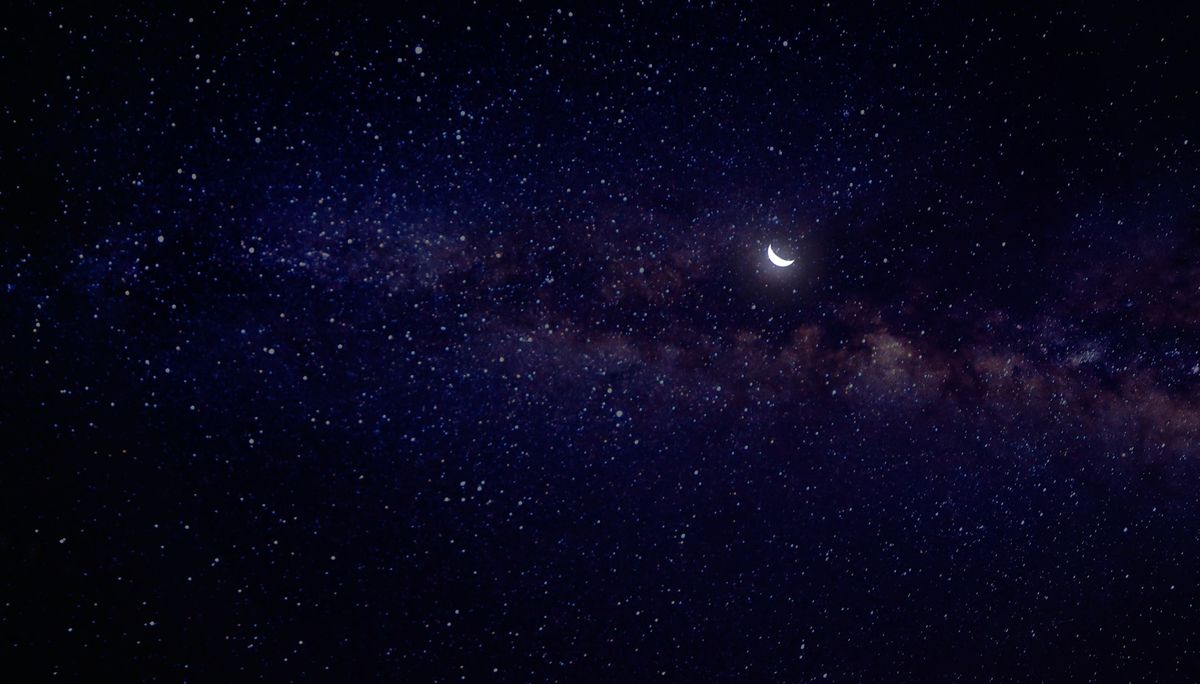
While putting together an understanding of the Stellar SDK and developing a platform for smart contracts (which I'll announce later); I'll be blogging excerpts of how to interact with the Stellar network through the JavaScript SDK as I discover more.
Currently, I'm working through a process of initializing an account on the network that behaves as a pure smart contract account (will be used for setting up access, rules and executing a binding contract on the public ledger). Initially, I felt it would make most logical sense to fund it after the user has decided on how the contract will execute and we can easily calculate fees and totals. However, due to some technical restrictions the account must be created first before being able to create and sign valid transaction envelopes (I'll make a follow up later).
The current user experience is:
- User lands on smart contract creation page
- A new keypair is generated and shown to the user
- The user is required to fund the account with 2 XLM
- Request the user enter their funding public key address
- A status indicator should display so the user knows we're watching and waiting for the payment
What I'm building
That's a bit long winded, but, outlines clearly what interfaces will be necessary for the code I'm about to share below.
Let's make the assumption that we have received the funding public key address from our user.
The user has sent a transaction over the network for our desired 2 XLM to initialize the smart contract.
We'd like to watch for this transaction over the Stellar network and we're in luck because the Stellar JavaScript SDK has a streaming capability.
import Stellar from 'stellar-sdk'
// Set the server
const server = new Stellar.Server('https://horizon-testnet.stellar.org')
Stellar.Network.useTestNetwork()
let funding_account = "" // This needs to be a valid account on the network and should return an Account object
let stream = server.payments()
.forAccount(funding_account)
.cursor('now')
.stream({
onmessage: function(message) {
if (message.type == "create_account" && message.account == app.contract.public_key) {
// Set your own code after we find our create account event
stream()
}
}
})
The code above will (in our case use the Test Network) take our input from our user and watch for a "create_account" event on that funding public key address. Since we now know this information, and our smart contract account public key we can further verify this is the proper individual interacting with our system.
The system will respond with the following JSON.
{"_links":
{"self":{"href":"https://horizon-testnet.stellar.org/operations/37862971392684033"},
"transaction":{"href":"https://horizon-testnet.stellar.org/transactions/0ad5c77ffe9796f4ac96e9536bf93e54e3641efe0f2a66d7a3be2f1340f86a39"},
"effects":{"href":"https://horizon-testnet.stellar.org/operations/37862971392684033/effects"},
"succeeds":{"href":"https://horizon-testnet.stellar.org/effects?order=desc\u0026cursor=37862971392684033"},
"precedes":{"href":"https://horizon-testnet.stellar.org/effects?order=asc\u0026cursor=37862971392684033"}},
"id":"37862971392684033",
"paging_token":"37862971392684033",
"source_account":"GDUKSHZ2JFC4AYMHWIQTSMSRWT2H2GDLH6BG35UAMZPIYKBIRUC5JK5C",
"type":"create_account",
"type_i":0,
"created_at":"2018-05-06T04:38:12Z",
"transaction_hash":"0ad5c77ffe9796f4ac96e9536bf93e54e3641efe0f2a66d7a3be2f1340f86a39",
"starting_balance":"2.0000000",
"funder":"GDUKSHZ2JFC4AYMHWIQTSMSRWT2H2GDLH6BG35UAMZPIYKBIRUC5JK5C",
"account":"GC4L3V7NKMAN5BG6DVHJZLGVGPRYLBCJOHHIDFLL6S7N3ORJTCDA25E4"
}
As you can see, we get a wealth of information back at our disposal to make further decisions.
If you have questions comment below. Also interested to see what you're working on.